Suggested Videos
Part 4 - Command window
Part 5 - Immediate window
Part 6 - Watch window
We discussed the basics of watch window in visual studio in Part 6. Please watch Part 6 before proceeding.
When the application is in debug mode and if we navigate to Debug - Windows - Watch, you will see that there are 4 watch windows.
Have you ever wondered the use of multiple watch windows?
Multiple watch windows are useful when you want to compare 2 object properties during debugging.
Let's understand their purpose with an example. Create a new console application with name = ConsoleApplication1. Copy and paste the following code.
Place a breakpoint on the foreach loop in the Main() method and run the application in debug mode. When the application execution stops at the break point, open 2 watch windows by going to Debug - Windows - Watch menu.
In Watch 1 window, type customer[0] in Name column and press Enter key. Along the same lines in Watch 2 window, type customer[1] in Name column and press Enter key. Notice that now you have the opportunity 2 inspect 2 customer object properties.
Is it possible to get a copy of the object from one watch window to another watch window.
Yes, simply drag and drop. If you change a property of the object in one watch window, the change will be automatically reflected in the other watch window.
Is it possible to replace the current object instance with a new object instance
Yes, simply create a new object instance in immediate window. For example the Watch 1 window below displays the first customer object in customers collection.
To replace the current Customer object in customers[0] location. Open Immediate window by going to Debug - Windows - Immediate. Type the following command in Immediate window and press Enter key.
customers[0] = new Customer()
Notice that the Watch 1 windows now displays, the default values of the new Customer object that we have just created.
Part 4 - Command window
Part 5 - Immediate window
Part 6 - Watch window
We discussed the basics of watch window in visual studio in Part 6. Please watch Part 6 before proceeding.
When the application is in debug mode and if we navigate to Debug - Windows - Watch, you will see that there are 4 watch windows.

Have you ever wondered the use of multiple watch windows?
Multiple watch windows are useful when you want to compare 2 object properties during debugging.
Let's understand their purpose with an example. Create a new console application with name = ConsoleApplication1. Copy and paste the following code.
using System;
using System.Collections.Generic;
namespace ConsoleApplication1
{
class Program
{
static void Main()
{
List<Customer>
customers = Customer.GetAllCustomers();
foreach (Customer customer in customers)
{
Console.WriteLine("First Name = {0} & Last Name = {1}",
customer.FirstName, customer.LastName);
}
}
}
class Customer
{
public int ID
{ get; set;
}
public string FirstName { get; set; }
public string LastName
{ get; set;
}
public string Gender
{ get; set;
}
public int Salary
{ get; set;
}
public static List<Customer> GetAllCustomers()
{
List<Customer>
listCustomers = new List<Customer>();
Customer customer1 = new Customer
{
ID = 101,
FirstName = "Mark",
LastName = "Scotts",
Gender = "Male",
Salary = 55000
};
Customer customer2 = new Customer
{
ID = 102,
FirstName = "Steve",
LastName = "Wicht",
Gender = "Male",
Salary = 45000
};
Customer customer3 = new Customer
{
ID = 103,
FirstName = "Pam",
LastName = "P",
Gender = "Female",
Salary = 65000
};
Customer customer4 = new Customer
{
ID = 104,
FirstName = "Todd",
LastName = "Snider",
Gender = "Male",
Salary = 45000
};
Customer customer5 = new Customer
{
ID = 105,
FirstName = "Valarie",
LastName = "Ward",
Gender = "Female",
Salary = 35000
};
listCustomers.Add(customer1);
listCustomers.Add(customer2);
listCustomers.Add(customer3);
listCustomers.Add(customer4);
listCustomers.Add(customer5);
return listCustomers;
}
}
}
Place a breakpoint on the foreach loop in the Main() method and run the application in debug mode. When the application execution stops at the break point, open 2 watch windows by going to Debug - Windows - Watch menu.
In Watch 1 window, type customer[0] in Name column and press Enter key. Along the same lines in Watch 2 window, type customer[1] in Name column and press Enter key. Notice that now you have the opportunity 2 inspect 2 customer object properties.

Is it possible to get a copy of the object from one watch window to another watch window.
Yes, simply drag and drop. If you change a property of the object in one watch window, the change will be automatically reflected in the other watch window.
Is it possible to replace the current object instance with a new object instance
Yes, simply create a new object instance in immediate window. For example the Watch 1 window below displays the first customer object in customers collection.

To replace the current Customer object in customers[0] location. Open Immediate window by going to Debug - Windows - Immediate. Type the following command in Immediate window and press Enter key.
customers[0] = new Customer()
Notice that the Watch 1 windows now displays, the default values of the new Customer object that we have just created.

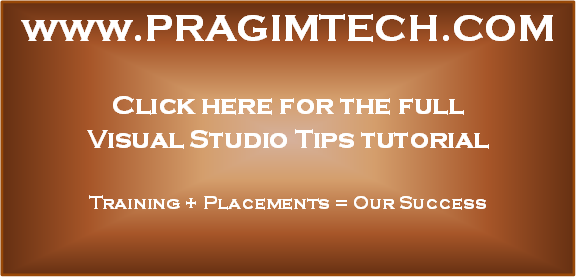
No comments:
Post a Comment
It would be great if you can help share these free resources