Suggested Videos
Part 34 - Duplex message exchange pattern in WCF
Part 35 - Sending large messages in WCF using MTOM
Part 36 - Instancing modes in WCF
In this video we will discuss, PerCall instance context mode in WCF with an example. This is continuation to Part 36. Please watch Part 36 before proceeding.
When the instance context mode for a wcf service is set to PerCall, a new instance of the wcf service object is created for every request, irrespective of whether the request comes from the same client or a different client. Let's prove this with an example.
PerCall instance context mode example:
Step 1: Create a class library project with name = SimpleService. Delete class1.cs file. Add a WCF service with name = SimpleService.
Step 2: Copy and paste the following code in ISimpleService.cs file
Step 3: Copy and paste the following code in SimpleService.cs file
Step 4: Right click on SimpleService solution in Solution Explorer and a Console Application with name = Host. We will use this project to host the WCF service.
Step 5: Right click on References folder under Host project in Solution Explorer, and a reference to SimpleService project and System.ServiceModel assembly.
Step 6: Copy and paste the following code in Program.cs file
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
Step 9: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 10: Now let's create a client for the WCF service. Create a new console application with name = Client
Step 11: Add a service reference to SimpleService. To do this, right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up SimpleService. In the Namespace textbox type SimpleService and click OK.
Step 12: Copy and paste the following code in Program.cs file in the client application.
Step 13: Run the application, and you should see the following output.
So, what's happening? Why is the number not incremented beyond 1?
The reason for this is simple. We have set instance context mode of the WCF service to PerCall. This means that, every time we call IncrementNumber() method from the client application,
1. A new instance of SimpleService is created
2. Private variable _number in the wcf service is initialized to ZERO. The method returns the incremented value 1 to the client and the service instance object is destroyed.
3. When a subsequent call is made either from the same client or different client, the above steps are repeated. Hence the number always stays at 1.
What are the implications of a PerCall WCF service?
1. Better memory usage as service objects are freed immediately after the method call returns
2. Concurrency not an issue
3. Application scalability is better
4. State not maintained between calls.
5. Performance could be an issue as there is overhead involved in reconstructing the service instance state on each and every method call.
Part 34 - Duplex message exchange pattern in WCF
Part 35 - Sending large messages in WCF using MTOM
Part 36 - Instancing modes in WCF
In this video we will discuss, PerCall instance context mode in WCF with an example. This is continuation to Part 36. Please watch Part 36 before proceeding.
When the instance context mode for a wcf service is set to PerCall, a new instance of the wcf service object is created for every request, irrespective of whether the request comes from the same client or a different client. Let's prove this with an example.
PerCall instance context mode example:
Step 1: Create a class library project with name = SimpleService. Delete class1.cs file. Add a WCF service with name = SimpleService.
Step 2: Copy and paste the following code in ISimpleService.cs file
using System.ServiceModel;
namespace SimpleService
{
[ServiceContract]
public interface ISimpleService
{
[OperationContract]
int IncrementNumber();
}
}
Step 3: Copy and paste the following code in SimpleService.cs file
using System.ServiceModel;
namespace SimpleService
{
// Set InstanceContextMode to PerCall
[ServiceBehavior(InstanceContextMode = InstanceContextMode.PerCall)]
public class SimpleService : ISimpleService
{
int _number;
public int IncrementNumber()
{
_number = _number + 1;
return _number;
}
}
}
Step 4: Right click on SimpleService solution in Solution Explorer and a Console Application with name = Host. We will use this project to host the WCF service.
Step 5: Right click on References folder under Host project in Solution Explorer, and a reference to SimpleService project and System.ServiceModel assembly.
Step 6: Copy and paste the following code in Program.cs file
using System;
using System.ServiceModel;
namespace Host
{
class Program
{
public static void Main()
{
using (ServiceHost host = new
ServiceHost(typeof(SimpleService.SimpleService)))
{
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
}
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehavior">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="mexBehavior" name="SimpleService.SimpleService">
<endpoint address="SimpleService" binding="netTcpBinding"
contract="SimpleService.ISimpleService" />
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080" />
<add baseAddress="net.tcp://localhost:8090" />
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 9: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 10: Now let's create a client for the WCF service. Create a new console application with name = Client
Step 11: Add a service reference to SimpleService. To do this, right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up SimpleService. In the Namespace textbox type SimpleService and click OK.
Step 12: Copy and paste the following code in Program.cs file in the client application.
using System;
namespace Client
{
class Program
{
public static void Main()
{
SimpleService.SimpleServiceClient
client =
new SimpleService.SimpleServiceClient();
int number = client.IncrementNumber();
Console.WriteLine("Number
after first call = " + number);
number = client.IncrementNumber();
Console.WriteLine("Number after second call = " +
number);
number = client.IncrementNumber();
Console.WriteLine("Number after third call = " +
number);
}
}
}
Step 13: Run the application, and you should see the following output.

So, what's happening? Why is the number not incremented beyond 1?
The reason for this is simple. We have set instance context mode of the WCF service to PerCall. This means that, every time we call IncrementNumber() method from the client application,
1. A new instance of SimpleService is created
2. Private variable _number in the wcf service is initialized to ZERO. The method returns the incremented value 1 to the client and the service instance object is destroyed.
3. When a subsequent call is made either from the same client or different client, the above steps are repeated. Hence the number always stays at 1.
What are the implications of a PerCall WCF service?
1. Better memory usage as service objects are freed immediately after the method call returns
2. Concurrency not an issue
3. Application scalability is better
4. State not maintained between calls.
5. Performance could be an issue as there is overhead involved in reconstructing the service instance state on each and every method call.
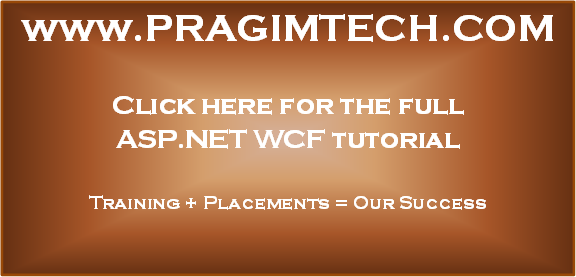
No comments:
Post a Comment
It would be great if you can help share these free resources