Suggested Videos
Part 1 - Introduction to WCF
In this video we will discuss creating a simple remoting and a web service. This is continuation to Part 1. Please watch Part 1 from WCF video tutorial before proceeding.
We have 2 clients and we need to implement a service a for them.
1. The first client is using a Java application to interact with our service, so for interoperability this client wants messages to be in XML format and the protocol to be HTTP.
2. The second client uses .NET, so for better performance this client wants messages formatted in binary over TCP protocol.
To satisfy the requirement of the first client let's create a web service. Web services use HTTP protocol and XML message format. So interoperability requirement of the first client will be met. Web services can be consumed by any client built on any platform.
To create the web service
1. Create an empty asp.net web application project and name it WebServicesDemo.
2. Right click on the project name in solution explorer and add a web service. Name it HelloWebService.
3. Copy and paste the following code HelloWebService.asmx.cs.
using System.Web.Services;
namespace WebServicesDemo
{
[WebService(Namespace = "http://pragimtech.com/WebServices")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class HelloWebService : System.Web.Services.WebService
{
[WebMethod]
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
Build the solution.
Creating a client for the Web Service.
1. Right click on WebServicesDemo solution in solution explorer and add a new asp.net empty web application project and name it HelloWebApplication.
2. Right click on References folder in HelloWebApplication project and select Add Service Reference option. In Add Service Reference dialog box click the Discover button. In the namespace textbox type HelloWebService and click OK. This should generate a proxy class to invoke the HelloWebService.
3. Right click on HelloWebApplication project name in solution explorer and a new web form. This should add WebForm1.aspx
4. Copy and paste the following HTML on WebForm1.aspx
<table style="font-family:Arial">
<tr>
<td>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<asp:Button ID="Button1" runat="server" Text="Get Message"
onclick="Button1_Click" />
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label1" runat="server" Font-Bold="true">
</asp:Label>
</td>
</tr>
</table>
5. Copy and paste the following code in WebForm1.aspx.cs
protected void Button1_Click(object sender, EventArgs e)
{
HelloWebService.HelloWebServiceSoapClient client = new
HelloWebService.HelloWebServiceSoapClient();
Label1.Text = client.GetMessage(TextBox1.Text);
}
The ASP.NET web application is now able to communicate with the web service. Not just asp.net, a JAVA application can also consume the web service.
To satisfy the requirement of the second client let's create a .NET remoting service.
Creating a remoting service
1. Create a new Class Library project and name it IHelloRemotingService.
2. Rename Class1.cs file to IHelloRemotingService.cs. Copy and paste the following code in IHelloRemotingService.cs file.
namespace IHelloRemotingService
{
public interface IHelloRemtingService
{
string GetMessage(string name);
}
}
3. Right click on IHelloRemotingService solution in solution explorer and add new class library project, and name it HelloRemotingService.
4. We want to use interface IHelloRemotingService in HelloRemotingService project. So add a reference to IHelloRemotingService project.
5. Rename Class1.cs file to HelloRemotingService.cs. Copy and paste the following code in HelloRemotingService.cs file.
using System;
namespace HelloRemotingService
{
public class HelloRemotingService : MarshalByRefObject,
IHelloRemotingService.IHelloRemtingService
{
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
6. Now we need to host the remoting service. To host it let's use a console application. A windows application or IIS can also be used to host the remoting service. Right click on IHelloRemotingService solution in solution explorer and add new Console Application project, and name it RemotingServiceHost.
7. Add a reference to IHelloRemotingService and HelloRemotingService projects and System.Runtime.Remoting assembly.
8. Copy and paste the following code in Program.cs file
using System;
using System.Runtime.Remoting;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting.Channels.Tcp;
namespace RemotingServiceHost
{
class Program
{
static void Main()
{
HelloRemotingService.HelloRemotingService remotingService =
new HelloRemotingService.HelloRemotingService();
TcpChannel channel = new TcpChannel(8080);
ChannelServices.RegisterChannel(channel);
RemotingConfiguration.RegisterWellKnownServiceType(
typeof(HelloRemotingService.HelloRemotingService), "GetMessage",
WellKnownObjectMode.Singleton);
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
9. Now we need to create the client for our remoting service. Let's use windows application as the client. Right click on IHelloRemotingService solution in solution explorer and add new windows application.
10. Add a reference to IHelloRemotingService project and System.Runtime.Remoting assembly.
11. Drag and drop a textbox, button and a label control on Form1 in the windows application.
12. Double click the button to generate the click event handler. Copy and paste the following code in Form1.cs.
using System;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting.Channels.Tcp;
using System.Windows.Forms;
namespace HelloRemotingServiceClient
{
public partial class Form1 : Form
{
IHelloRemotingService.IHelloRemtingService client;
public Form1()
{
InitializeComponent();
TcpChannel channel = new TcpChannel();
ChannelServices.RegisterChannel(channel);
client = (IHelloRemotingService.IHelloRemtingService)Activator.GetObject(
typeof(IHelloRemotingService.IHelloRemtingService),
"tcp://localhost:8080/GetMessage");
}
private void button1_Click(object sender, EventArgs e)
{
label1.Text = client.GetMessage(textBox1.Text);
}
}
}
By this point you may have already realized how different web service and remoting programming models are. In Part 3, we will discuss implementing a single WCF service that can satisfy the requirements of both the clients.
Can we use .NET Remoting to build a Web service?
Yes.
If the client is not built using .NET platform, will they be able to consume a .NET Remoting web service?
They can, but we need to be very careful in choosing the data types that we use in the service, and client-activated objects and events should be avoided. But keep in mind .NET Remoting is not meant for implementing interoperable services.
If your goal is to build interoperable services use ASP.NET Web Services. But with introduction of WCF, both .NET Remoting and ASP.NET Web Services are legacy technologies.
Part 1 - Introduction to WCF
In this video we will discuss creating a simple remoting and a web service. This is continuation to Part 1. Please watch Part 1 from WCF video tutorial before proceeding.
We have 2 clients and we need to implement a service a for them.
1. The first client is using a Java application to interact with our service, so for interoperability this client wants messages to be in XML format and the protocol to be HTTP.
2. The second client uses .NET, so for better performance this client wants messages formatted in binary over TCP protocol.
To satisfy the requirement of the first client let's create a web service. Web services use HTTP protocol and XML message format. So interoperability requirement of the first client will be met. Web services can be consumed by any client built on any platform.
To create the web service
1. Create an empty asp.net web application project and name it WebServicesDemo.
2. Right click on the project name in solution explorer and add a web service. Name it HelloWebService.
3. Copy and paste the following code HelloWebService.asmx.cs.
using System.Web.Services;
namespace WebServicesDemo
{
[WebService(Namespace = "http://pragimtech.com/WebServices")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class HelloWebService : System.Web.Services.WebService
{
[WebMethod]
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
Build the solution.
Creating a client for the Web Service.
1. Right click on WebServicesDemo solution in solution explorer and add a new asp.net empty web application project and name it HelloWebApplication.
2. Right click on References folder in HelloWebApplication project and select Add Service Reference option. In Add Service Reference dialog box click the Discover button. In the namespace textbox type HelloWebService and click OK. This should generate a proxy class to invoke the HelloWebService.
3. Right click on HelloWebApplication project name in solution explorer and a new web form. This should add WebForm1.aspx
4. Copy and paste the following HTML on WebForm1.aspx
<table style="font-family:Arial">
<tr>
<td>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<asp:Button ID="Button1" runat="server" Text="Get Message"
onclick="Button1_Click" />
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label1" runat="server" Font-Bold="true">
</asp:Label>
</td>
</tr>
</table>
5. Copy and paste the following code in WebForm1.aspx.cs
protected void Button1_Click(object sender, EventArgs e)
{
HelloWebService.HelloWebServiceSoapClient client = new
HelloWebService.HelloWebServiceSoapClient();
Label1.Text = client.GetMessage(TextBox1.Text);
}
The ASP.NET web application is now able to communicate with the web service. Not just asp.net, a JAVA application can also consume the web service.
To satisfy the requirement of the second client let's create a .NET remoting service.
Creating a remoting service
1. Create a new Class Library project and name it IHelloRemotingService.
2. Rename Class1.cs file to IHelloRemotingService.cs. Copy and paste the following code in IHelloRemotingService.cs file.
namespace IHelloRemotingService
{
public interface IHelloRemtingService
{
string GetMessage(string name);
}
}
3. Right click on IHelloRemotingService solution in solution explorer and add new class library project, and name it HelloRemotingService.
4. We want to use interface IHelloRemotingService in HelloRemotingService project. So add a reference to IHelloRemotingService project.
5. Rename Class1.cs file to HelloRemotingService.cs. Copy and paste the following code in HelloRemotingService.cs file.
using System;
namespace HelloRemotingService
{
public class HelloRemotingService : MarshalByRefObject,
IHelloRemotingService.IHelloRemtingService
{
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
6. Now we need to host the remoting service. To host it let's use a console application. A windows application or IIS can also be used to host the remoting service. Right click on IHelloRemotingService solution in solution explorer and add new Console Application project, and name it RemotingServiceHost.
7. Add a reference to IHelloRemotingService and HelloRemotingService projects and System.Runtime.Remoting assembly.
8. Copy and paste the following code in Program.cs file
using System;
using System.Runtime.Remoting;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting.Channels.Tcp;
namespace RemotingServiceHost
{
class Program
{
static void Main()
{
HelloRemotingService.HelloRemotingService remotingService =
new HelloRemotingService.HelloRemotingService();
TcpChannel channel = new TcpChannel(8080);
ChannelServices.RegisterChannel(channel);
RemotingConfiguration.RegisterWellKnownServiceType(
typeof(HelloRemotingService.HelloRemotingService), "GetMessage",
WellKnownObjectMode.Singleton);
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
9. Now we need to create the client for our remoting service. Let's use windows application as the client. Right click on IHelloRemotingService solution in solution explorer and add new windows application.
10. Add a reference to IHelloRemotingService project and System.Runtime.Remoting assembly.
11. Drag and drop a textbox, button and a label control on Form1 in the windows application.
12. Double click the button to generate the click event handler. Copy and paste the following code in Form1.cs.
using System;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting.Channels.Tcp;
using System.Windows.Forms;
namespace HelloRemotingServiceClient
{
public partial class Form1 : Form
{
IHelloRemotingService.IHelloRemtingService client;
public Form1()
{
InitializeComponent();
TcpChannel channel = new TcpChannel();
ChannelServices.RegisterChannel(channel);
client = (IHelloRemotingService.IHelloRemtingService)Activator.GetObject(
typeof(IHelloRemotingService.IHelloRemtingService),
"tcp://localhost:8080/GetMessage");
}
private void button1_Click(object sender, EventArgs e)
{
label1.Text = client.GetMessage(textBox1.Text);
}
}
}
By this point you may have already realized how different web service and remoting programming models are. In Part 3, we will discuss implementing a single WCF service that can satisfy the requirements of both the clients.
Can we use .NET Remoting to build a Web service?
Yes.
If the client is not built using .NET platform, will they be able to consume a .NET Remoting web service?
They can, but we need to be very careful in choosing the data types that we use in the service, and client-activated objects and events should be avoided. But keep in mind .NET Remoting is not meant for implementing interoperable services.
If your goal is to build interoperable services use ASP.NET Web Services. But with introduction of WCF, both .NET Remoting and ASP.NET Web Services are legacy technologies.
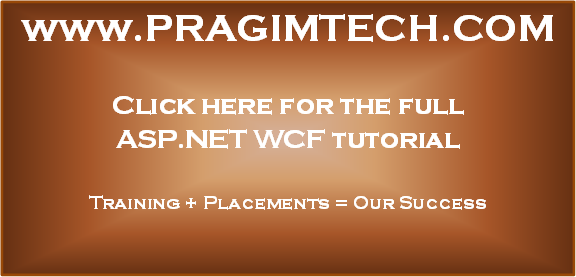
What is a proxy ??? ... please explain in detail .!!
ReplyDeleteAn unhandled exception of type 'System.Net.Sockets.SocketException' occurred in mscorlib.dll
ReplyDeletePlease help to solve this error....
Make sure your service would be running first before trying to consume it.Run the exe file of the console application first and then run the win form client file while the service would already be up
DeleteHello sir,
ReplyDeleteThis is the error that we got
SocketException was unhandled!
No connection could be made because the target machine actively refused it 127.0.0.1:8080
Plz Help.
When you follow all the steps exactly in this video, there are no problems.
DeleteDear Sir,
ReplyDeleteWhat if the "HelloWebServiceSoapClient" cant be resolved in the client for the WebService.
Do you have any hint?
Thanks and best regards
An unhandled exception of type 'System.Runtime.Remoting.RemotingException' occurred in mscorlib.dll
ReplyDeleteAdditional information: Tcp channel protocol violation: expecting preamble.
Hivenkat,
ReplyDeletePlease explain What is proxy? what is its puprose in WCF?
Hi Sir,
ReplyDeletePlease Make the WEB API blog.
I don´t know why I´m getting this error
ReplyDeleteC:\Palo IT\WCF\IHelloRemotingService\RemotingServiceHost\bin\Debug>RemotingServiceHost.exe
Excepción no controlada: System.Net.Sockets.SocketException: Intento de acceso a un socket no permitido por sus permisos de acceso
en System.Net.Sockets.Socket.DoBind(EndPoint endPointSnapshot, SocketAddress socketAddress)
en System.Net.Sockets.Socket.Bind(EndPoint localEP)
en System.Net.Sockets.TcpListener.Start(Int32 backlog)
en System.Runtime.Remoting.Channels.ExclusiveTcpListener.Start(Boolean exclusiveAddressUse)
en System.Runtime.Remoting.Channels.Tcp.TcpServerChannel.StartListening(Object data)
en System.Runtime.Remoting.Channels.Tcp.TcpServerChannel.SetupChannel()
en System.Runtime.Remoting.Channels.Tcp.TcpServerChannel..ctor(Int32 port)
en System.Runtime.Remoting.Channels.Tcp.TcpChannel..ctor(Int32 port)
en RemotingServiceHost.Program.Main() en C:\Palo IT\WCF\IHelloRemotingService\RemotingServiceHost\Program.cs:línea 13