Suggested Videos
Part 148 - Passing data from content page to master page
Part 149 - Passing data from master page to content page
Part 150 - Default content in contentplaceholder of a master page
In this video, we will discuss, how to select a master page dynamically at runtime. Let us understand this with an example.
This is what we want to achieve.
1. We have 2 master pages in our application.
2. Include a dropdownlist on both the master pages. The dropdownlist should display both the master pages.
3. As we select a master page from the dropdownlist, we want the selected master page to be dynamically set as the master page.
Here are the steps:
1. Create a new asp.net webforms application and name it WebFormsDemo.
2. Add a class file to the project and name it BaseMaterPage.cs. Copy and paste the following code. Notice that the BaseMaterPage class inherits from System.Web.UI.MasterPage. This BaseMaterPage class will be used as the base class for the 2 master pages that we will be creating later.
using System.Web.UI.WebControls;
namespace WebFormsDemo
{
public abstract class BaseMaterPage : System.Web.UI.MasterPage
{
public abstract Panel SearchPanel { get; }
public abstract string SearchTerm { get; }
public abstract Button SearchButton { get; }
}
}
3. Add a master page and name it Site.Master. Copy and paste the following html.
<table style="font-family: Arial">
<tr>
<td colspan="2" style="width: 800px; height: 80px; text-align:
center; background-color: #BDBDBD;">
<h1>WebSite Header</h1>
<asp:Panel ID="panelSearch" runat="server">
<b>Search : </b>
<asp:TextBox ID="txtSerach" runat="server"></asp:TextBox>
<asp:Button ID="btnSearch" runat="server" Text="Search" />
</asp:Panel>
</td>
</tr>
<tr>
<td style="height: 500px; background-color: #D8D8D8; width: 150px">
<b>Select Master Page</b>
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="true"
OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Text="Master Page 1" Value="~/Site.Master">
</asp:ListItem>
<asp:ListItem Text="Master Page 2" Value="~/Site2.Master">
</asp:ListItem>
</asp:DropDownList>
</td>
<td style="height: 500px; background-color: #E6E6E6; width: 650px">
<asp:ContentPlaceHolder ID="MainContent" runat="server">
<h1>
Section that changes on a page by page basis</h1>
</asp:ContentPlaceHolder>
</td>
</tr>
<tr>
<td colspan="2" style="background-color: #BDBDBD; text-align: center">
<b>Website Footer</b>
</td>
</tr>
</table>
4. Copy and paste the following code in the code behind file. Notice that the Site master page class inherits from the BaseMaterPage class that we created in Step 1.
public partial class Site : BaseMaterPage
{
protected void Page_Load(object sender, EventArgs e)
{
}
public override Panel SearchPanel
{
get
{
return panelSearch;
}
}
public override string SearchTerm
{
get
{
return txtSerach.Text;
}
}
public override Button SearchButton
{
get
{
return btnSearch;
}
}
protected void DropDownList1_SelectedIndexChanged
(object sender, EventArgs e)
{
Session["SELECTED_MASTERPAGE"] = DropDownList1.SelectedValue;
Response.Redirect(Request.Url.AbsoluteUri);
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
DropDownList1.SelectedValue =
Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
5. Add another master page and name it Site2.Master. Copy and paste the following html.
<table style="font-family: Arial; color:White">
<tr>
<td colspan="2" style="width: 800px; height: 80px;
text-align: center; background-color:Red;">
<h1>WebSite Header</h1>
<asp:Panel ID="panelSearch" runat="server">
<b>Search : </b>
<asp:TextBox ID="txtSerach" runat="server"></asp:TextBox>
<asp:Button ID="btnSearch" runat="server" Text="Search"/>
</asp:Panel>
</td>
</tr>
<tr>
<td style="height: 500px; background-color:Green; width: 650px">
<asp:ContentPlaceHolder ID="MainContent" runat="server">
<h1>Section that changes on a page by page basis</h1>
</asp:ContentPlaceHolder>
</td>
<td style="height: 500px; background-color:Blue; width: 150px">
<b>Select Master Page</b>
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="true"
OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Text="Master Page 1" Value="~/Site.Master">
</asp:ListItem>
<asp:ListItem Text="Master Page 2" Value="~/Site2.Master">
</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td colspan="2" style="background-color:Red; text-align: center">
<b>Website Footer</b>
</td>
</tr>
</table>
6. Copy and paste the following code in the code behind file. Notice that the Site2 master page class inherits from the BaseMaterPage class that we created in Step 1.
public partial class Site2 : BaseMaterPage
{
protected void Page_Load(object sender, EventArgs e)
{
}
public override Panel SearchPanel
{
get
{
return panelSearch;
}
}
public override string SearchTerm
{
get
{
return txtSerach.Text;
}
}
public override Button SearchButton
{
get
{
return btnSearch;
}
}
protected void DropDownList1_SelectedIndexChanged
(object sender, EventArgs e)
{
Session["SELECTED_MASTERPAGE"] = DropDownList1.SelectedValue;
Response.Redirect(Request.Url.AbsoluteUri);
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
DropDownList1.SelectedValue =
Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
7. Add a class file and name it BasePage.cs. Copy and paste the following code. Notice that the BasePage class inherits from System.Web.UI.Page. This BasePage class will be used as the base class for all the content pages in our application.
using System;
namespace WebFormsDemo
{
public class BasePage : System.Web.UI.Page
{
protected override void OnPreInit(EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
this.MasterPageFile = Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
}
8. Right click on Site.Master page and select Add Content Page. Copy and paste the following html. Notice that we have set MasterType to BaseMaterPage class.
<%@ Page Title="" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="WebFormsDemo.WebForm1" %>
<%@ MasterType TypeName="WebFormsDemo.BaseMaterPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="MainContent" runat="server">
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</asp:Content>
9. In the code-behind file of the content page, copy and paste the following code.
public partial class WebForm1 : BasePage
{
protected void Page_Init(object sender, EventArgs e)
{
Master.SearchButton.Click += new
EventHandler(SearchButton_Click);
}
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GetData(null);
}
}
protected void SearchButton_Click(object sender, EventArgs e)
{
GetData(Master.SearchTerm);
}
private void GetData(string searchTerm)
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"]
.ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spSearch", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter searchParameter = new SqlParameter
("@SearchTerm", searchTerm ?? string.Empty);
cmd.Parameters.Add(searchParameter);
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
}
}
}
Note: Please include the following USING declarations
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
If you need the sql script to create and populate table tblStudents, please refer to Part 149. The T-SQL for stored procedure spSearch is also available in Part 149.
Part 148 - Passing data from content page to master page
Part 149 - Passing data from master page to content page
Part 150 - Default content in contentplaceholder of a master page
In this video, we will discuss, how to select a master page dynamically at runtime. Let us understand this with an example.
This is what we want to achieve.
1. We have 2 master pages in our application.
2. Include a dropdownlist on both the master pages. The dropdownlist should display both the master pages.
3. As we select a master page from the dropdownlist, we want the selected master page to be dynamically set as the master page.

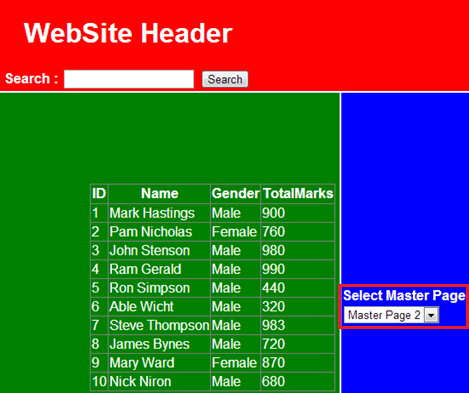
Here are the steps:
1. Create a new asp.net webforms application and name it WebFormsDemo.
2. Add a class file to the project and name it BaseMaterPage.cs. Copy and paste the following code. Notice that the BaseMaterPage class inherits from System.Web.UI.MasterPage. This BaseMaterPage class will be used as the base class for the 2 master pages that we will be creating later.
using System.Web.UI.WebControls;
namespace WebFormsDemo
{
public abstract class BaseMaterPage : System.Web.UI.MasterPage
{
public abstract Panel SearchPanel { get; }
public abstract string SearchTerm { get; }
public abstract Button SearchButton { get; }
}
}
3. Add a master page and name it Site.Master. Copy and paste the following html.
<table style="font-family: Arial">
<tr>
<td colspan="2" style="width: 800px; height: 80px; text-align:
center; background-color: #BDBDBD;">
<h1>WebSite Header</h1>
<asp:Panel ID="panelSearch" runat="server">
<b>Search : </b>
<asp:TextBox ID="txtSerach" runat="server"></asp:TextBox>
<asp:Button ID="btnSearch" runat="server" Text="Search" />
</asp:Panel>
</td>
</tr>
<tr>
<td style="height: 500px; background-color: #D8D8D8; width: 150px">
<b>Select Master Page</b>
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="true"
OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Text="Master Page 1" Value="~/Site.Master">
</asp:ListItem>
<asp:ListItem Text="Master Page 2" Value="~/Site2.Master">
</asp:ListItem>
</asp:DropDownList>
</td>
<td style="height: 500px; background-color: #E6E6E6; width: 650px">
<asp:ContentPlaceHolder ID="MainContent" runat="server">
<h1>
Section that changes on a page by page basis</h1>
</asp:ContentPlaceHolder>
</td>
</tr>
<tr>
<td colspan="2" style="background-color: #BDBDBD; text-align: center">
<b>Website Footer</b>
</td>
</tr>
</table>
4. Copy and paste the following code in the code behind file. Notice that the Site master page class inherits from the BaseMaterPage class that we created in Step 1.
public partial class Site : BaseMaterPage
{
protected void Page_Load(object sender, EventArgs e)
{
}
public override Panel SearchPanel
{
get
{
return panelSearch;
}
}
public override string SearchTerm
{
get
{
return txtSerach.Text;
}
}
public override Button SearchButton
{
get
{
return btnSearch;
}
}
protected void DropDownList1_SelectedIndexChanged
(object sender, EventArgs e)
{
Session["SELECTED_MASTERPAGE"] = DropDownList1.SelectedValue;
Response.Redirect(Request.Url.AbsoluteUri);
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
DropDownList1.SelectedValue =
Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
5. Add another master page and name it Site2.Master. Copy and paste the following html.
<table style="font-family: Arial; color:White">
<tr>
<td colspan="2" style="width: 800px; height: 80px;
text-align: center; background-color:Red;">
<h1>WebSite Header</h1>
<asp:Panel ID="panelSearch" runat="server">
<b>Search : </b>
<asp:TextBox ID="txtSerach" runat="server"></asp:TextBox>
<asp:Button ID="btnSearch" runat="server" Text="Search"/>
</asp:Panel>
</td>
</tr>
<tr>
<td style="height: 500px; background-color:Green; width: 650px">
<asp:ContentPlaceHolder ID="MainContent" runat="server">
<h1>Section that changes on a page by page basis</h1>
</asp:ContentPlaceHolder>
</td>
<td style="height: 500px; background-color:Blue; width: 150px">
<b>Select Master Page</b>
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="true"
OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Text="Master Page 1" Value="~/Site.Master">
</asp:ListItem>
<asp:ListItem Text="Master Page 2" Value="~/Site2.Master">
</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td colspan="2" style="background-color:Red; text-align: center">
<b>Website Footer</b>
</td>
</tr>
</table>
6. Copy and paste the following code in the code behind file. Notice that the Site2 master page class inherits from the BaseMaterPage class that we created in Step 1.
public partial class Site2 : BaseMaterPage
{
protected void Page_Load(object sender, EventArgs e)
{
}
public override Panel SearchPanel
{
get
{
return panelSearch;
}
}
public override string SearchTerm
{
get
{
return txtSerach.Text;
}
}
public override Button SearchButton
{
get
{
return btnSearch;
}
}
protected void DropDownList1_SelectedIndexChanged
(object sender, EventArgs e)
{
Session["SELECTED_MASTERPAGE"] = DropDownList1.SelectedValue;
Response.Redirect(Request.Url.AbsoluteUri);
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
DropDownList1.SelectedValue =
Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
7. Add a class file and name it BasePage.cs. Copy and paste the following code. Notice that the BasePage class inherits from System.Web.UI.Page. This BasePage class will be used as the base class for all the content pages in our application.
using System;
namespace WebFormsDemo
{
public class BasePage : System.Web.UI.Page
{
protected override void OnPreInit(EventArgs e)
{
if (Session["SELECTED_MASTERPAGE"] != null)
{
this.MasterPageFile = Session["SELECTED_MASTERPAGE"].ToString();
}
}
}
}
8. Right click on Site.Master page and select Add Content Page. Copy and paste the following html. Notice that we have set MasterType to BaseMaterPage class.
<%@ Page Title="" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="WebFormsDemo.WebForm1" %>
<%@ MasterType TypeName="WebFormsDemo.BaseMaterPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="MainContent" runat="server">
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</asp:Content>
9. In the code-behind file of the content page, copy and paste the following code.
public partial class WebForm1 : BasePage
{
protected void Page_Init(object sender, EventArgs e)
{
Master.SearchButton.Click += new
EventHandler(SearchButton_Click);
}
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GetData(null);
}
}
protected void SearchButton_Click(object sender, EventArgs e)
{
GetData(Master.SearchTerm);
}
private void GetData(string searchTerm)
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"]
.ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spSearch", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter searchParameter = new SqlParameter
("@SearchTerm", searchTerm ?? string.Empty);
cmd.Parameters.Add(searchParameter);
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
}
}
}
Note: Please include the following USING declarations
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
If you need the sql script to create and populate table tblStudents, please refer to Part 149. The T-SQL for stored procedure spSearch is also available in Part 149.

Hi
ReplyDeleteI am getting Error "Make sure that the class defined in this code file matches the 'inherits' attribute, and that it extends the correct base class (e.g. Page or UserControl)." Please Help
i want to create aspx page dynamically from my website online...
ReplyDeletehow can i Do it??