Suggested Videos
Part 94 - Providing visual feedback using LoadingElementId AjaxOption
Part 95 - OnBegin, OnComplete, OnSuccess and OnFailure properties of AjaxOptions class
Part 96 - LoadingElementDuration property of AjaxOptions class
In this video, we will discuss implementing auto-complete functionality in an asp.net mvc application using jQuery Autocomplete Widget
Step 1: We will be using tblStudents table in this demo. Please find the sql script below, to create and populate this table with some data.
Create Table tblStudents
(
ID int identity primary key,
Name nvarchar(50),
Gender nvarchar(20),
TotalMarks int
)
Insert into tblStudents values('Mark Hastings','Male',900)
Insert into tblStudents values('Pam Nicholas','Female',760)
Insert into tblStudents values('John Stenson','Male',980)
Insert into tblStudents values('Ram Gerald','Male',990)
Insert into tblStudents values('Ron Simpson','Male',440)
Insert into tblStudents values('Able Wicht','Male',320)
Insert into tblStudents values('Steve Thompson','Male',983)
Insert into tblStudents values('James Bynes','Male',720)
Insert into tblStudents values('Mary Ward','Female',870)
Insert into tblStudents values('Nick Niron','Male',680)
Step 2: Create an ado.net entity data model using table tblStudents. Change the name of the generated entity from tblStudent to Student.
Step 3: Download autocomplete widget from http://jqueryui.com/download. The following folders and files will be downloded.
Step 4: Open "js" folder copy "jquery-1.9.1.js" and "jquery-ui-1.10.3.custom.min.js" files and paste them in the "Scripts" folder of you mvc project. Now open "css" folder. This folder will be present in "ui-lightness" folder. Copy "images" folder and "jquery-ui-1.10.3.custom.min.css" file and paste them in "Content" folder in your mvc project. If you are following along, at this point your solution explorer should look as shown below.
Step 5: Right click on the "Controllers" folder and add "Home" controller. Copy and paste the following code. Please make sure to include "MVCDemo.Models" namespace.
public class HomeController : Controller
{
public ActionResult Index()
{
DatabaseContext db = new DatabaseContext();
return View(db.Students);
}
[HttpPost]
public ActionResult Index(string searchTerm)
{
DatabaseContext db = new DatabaseContext();
List<Student> students;
if (string.IsNullOrEmpty(searchTerm))
{
students = db.Students.ToList();
}
else
{
students = db.Students
.Where(s => s.Name.StartsWith(searchTerm)).ToList();
}
return View(students);
}
public JsonResult GetStudents(string term)
{
DatabaseContext db = new DatabaseContext();
List<string> students = db.Students.Where(s => s.Name.StartsWith(term))
.Select(x => x.Name).ToList();
return Json(students, JsonRequestBehavior.AllowGet);
}
}
Step 6: Right click on the "Index" action method in the "HomeController" and add "Index" view. Copy and paste the following code.
@model IEnumerable<MVCDemo.Models.Student>
<link href="~/Content/jquery-ui-1.10.3.custom.min.css" rel="stylesheet" type="text/css" />
<script src="~/Scripts/jquery-1.9.1.js" type="text/javascript"></script>
<script src="~/Scripts/jquery-ui-1.10.3.custom.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(function () {
$("#txtSearch").autocomplete({
source: '@Url.Action("GetStudents")',
minLength: 1
});
});
</script>
<div style="font-family:Arial">
@using (@Html.BeginForm())
{
<b>Name: </b>
@Html.TextBox("searchTerm", null, new { id = "txtSearch" })
<input type="submit" value="Search" />
}
<table border="1">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Gender)
</th>
<th>
@Html.DisplayNameFor(model => model.TotalMarks)
</th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.TotalMarks)
</td>
</tr>
}
</table>
</div>
Part 94 - Providing visual feedback using LoadingElementId AjaxOption
Part 95 - OnBegin, OnComplete, OnSuccess and OnFailure properties of AjaxOptions class
Part 96 - LoadingElementDuration property of AjaxOptions class
In this video, we will discuss implementing auto-complete functionality in an asp.net mvc application using jQuery Autocomplete Widget
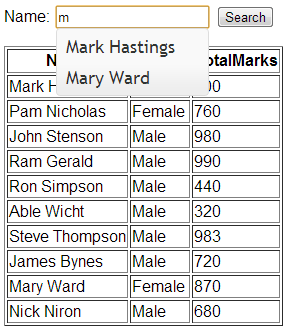
Step 1: We will be using tblStudents table in this demo. Please find the sql script below, to create and populate this table with some data.
Create Table tblStudents
(
ID int identity primary key,
Name nvarchar(50),
Gender nvarchar(20),
TotalMarks int
)
Insert into tblStudents values('Mark Hastings','Male',900)
Insert into tblStudents values('Pam Nicholas','Female',760)
Insert into tblStudents values('John Stenson','Male',980)
Insert into tblStudents values('Ram Gerald','Male',990)
Insert into tblStudents values('Ron Simpson','Male',440)
Insert into tblStudents values('Able Wicht','Male',320)
Insert into tblStudents values('Steve Thompson','Male',983)
Insert into tblStudents values('James Bynes','Male',720)
Insert into tblStudents values('Mary Ward','Female',870)
Insert into tblStudents values('Nick Niron','Male',680)
Step 2: Create an ado.net entity data model using table tblStudents. Change the name of the generated entity from tblStudent to Student.
Step 3: Download autocomplete widget from http://jqueryui.com/download. The following folders and files will be downloded.

Step 4: Open "js" folder copy "jquery-1.9.1.js" and "jquery-ui-1.10.3.custom.min.js" files and paste them in the "Scripts" folder of you mvc project. Now open "css" folder. This folder will be present in "ui-lightness" folder. Copy "images" folder and "jquery-ui-1.10.3.custom.min.css" file and paste them in "Content" folder in your mvc project. If you are following along, at this point your solution explorer should look as shown below.

Step 5: Right click on the "Controllers" folder and add "Home" controller. Copy and paste the following code. Please make sure to include "MVCDemo.Models" namespace.
public class HomeController : Controller
{
public ActionResult Index()
{
DatabaseContext db = new DatabaseContext();
return View(db.Students);
}
[HttpPost]
public ActionResult Index(string searchTerm)
{
DatabaseContext db = new DatabaseContext();
List<Student> students;
if (string.IsNullOrEmpty(searchTerm))
{
students = db.Students.ToList();
}
else
{
students = db.Students
.Where(s => s.Name.StartsWith(searchTerm)).ToList();
}
return View(students);
}
public JsonResult GetStudents(string term)
{
DatabaseContext db = new DatabaseContext();
List<string> students = db.Students.Where(s => s.Name.StartsWith(term))
.Select(x => x.Name).ToList();
return Json(students, JsonRequestBehavior.AllowGet);
}
}
Step 6: Right click on the "Index" action method in the "HomeController" and add "Index" view. Copy and paste the following code.
@model IEnumerable<MVCDemo.Models.Student>
<link href="~/Content/jquery-ui-1.10.3.custom.min.css" rel="stylesheet" type="text/css" />
<script src="~/Scripts/jquery-1.9.1.js" type="text/javascript"></script>
<script src="~/Scripts/jquery-ui-1.10.3.custom.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(function () {
$("#txtSearch").autocomplete({
source: '@Url.Action("GetStudents")',
minLength: 1
});
});
</script>
<div style="font-family:Arial">
@using (@Html.BeginForm())
{
<b>Name: </b>
@Html.TextBox("searchTerm", null, new { id = "txtSearch" })
<input type="submit" value="Search" />
}
<table border="1">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Gender)
</th>
<th>
@Html.DisplayNameFor(model => model.TotalMarks)
</th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.TotalMarks)
</td>
</tr>
}
</table>
</div>
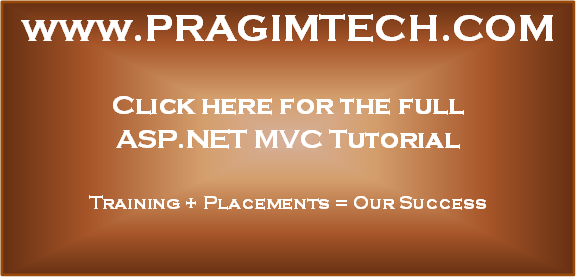
how to do autocomplete for all textbox.
ReplyDeletefor example: for name, address, city, etc...
I like your tutorials very much. they are very helpful.
Hi,
ReplyDeleteI am Using MVC3
it working with search button but not working autocomplete feature.
its not hitting GetStudents method.
see youtube comment....i have made few changes...and it work perfectly fine
DeleteI'm having the same issue as you too. I'm using MVC4 - Basic, but I'm not hitting GetStudents method. The only difference is that I'm using the latest jQueryui: 1.10.4 and instead of linq Startswith(), I'm using linq Contains() I'm using the exact folder structure as your picture above.
DeleteAm I missing something?
if u want to invoke the GetStudent() then do just write
Delete@using (@Html.BeginForm("GetStudents","Home"))
{//Getstudents is the name of method
//and Home is the name of controller
...remaining code put as it is ..!!
}
Hi,
ReplyDeletelike Gitu Digal I am interested in how to do autocomplete for more than one textbox, but in dependence of each other.
For example: the field name depends on company, address depends on name.
How can you achieve this?
Hi Gitu Digal, I'm also having same issue with GetStudents method. I am getting a blank dropdownlist for the search items. My search works fine when I enter first few characters and click on search button. I am using I'm the latest jQueryui: 1.10.4
ReplyDeleteAutocomplete is not working .Followed all the steps as detailed.
ReplyDeletecomment the code in this method --->this method id in Binddleconfig class-->> public static void RegisterBundles(BundleCollection bundles)
DeleteThen it will work fine
Greate sir working perfectly
ReplyDeleteHi Sir, Your videos are awesome.
ReplyDeleteCan you please explain the videos how to generate the parametrized reports using SSRS in mvc.
can you post your project here .. i did everything but still i can't get the autocomplete working ??
ReplyDeleteI did this and it started working for me.
Delete> for Mvc Artitecture you must delete already imbended
> @Scripts.Render("~/bundles/Jquery") and
> @Scripts.Render("~/bundles/Jquaryval") on all .cshtml files at the
> end and for also views/Shared/_layout.cshtml at the end and put our
> jaquery suitable files on his suitables .cshtmls files in head...and
> lets enjoy. put on head..these files
Thanks Venkat. It's a great effort by you and lots of people have been benefited (including me!!) by your excellent work. While working on this example one good friend of mine found the solution for addressing the issue of "autocomplete feature not hitting GetStudents method".
ReplyDeleteFollowing is the complete code.
$(function () {
$("#txtSearch").autocomplete({
source: '@Url.Action("GetStudents")',
minLength: 1,
response: function (event, ui) {
if (ui.content.length === 0) {
$("#lblMessage").text("No results found");
} else {
$("#lblMessage").empty();
}
}
});
});
Hi Thank you for such detail tutorials. Like other people around here, is there any way to look for more than one field at a time. So for example instead of having the full name, it would be first then last. But what ever the person enters it will show a match on first or last name
ReplyDeletehi,venkat please give an example for login based on roles
ReplyDeleteSir
ReplyDeleteI am Using MVC4
it working with search button but not working autocomplete feature.
jquery-ui-1.10.3.custom.min.css:1 Failed to load resource: the server responded with a status of 404 (Not Found)
jquery-1.9.1.js:1 Failed to load resource: the server responded with a status of 404 (Not Found)
jquery-ui-1.10.3.custom.min.js:1 Failed to load resource: the server responded with a status of 404 (Not Found)
jquery-ui-1.10.3.custom.min.css:1 Failed to load resource: the server responded with a status of 404 (Not Found)
jquery-1.9.1.js:1 Failed to load resource: the server responded with a status of 404 (Not Found)
jquery-ui-1.10.3.custom.min.js:1 Failed to load resource: the server responded with a status of 404 (Not Found)
Index:168 Uncaught TypeError: $(...).autocomplete is not a function
at HTMLDocument. (Index:168)
at c (jquery-1.10.2.min.js:21)
at Object.fireWith [as resolveWith] (jquery-1.10.2.min.js:21)
at Function.ready (jquery-1.10.2.min.js:21)
at HTMLDocument.q (jquery-1.10.2.min.js:21)
jquery-ui-1.10.3.custom.min.css:1 Failed to load resource: the server responded with a status of 404 (Not Found)