Suggested Videos
Part 62 - Implementing search functionality
Part 63 - Implement paging
Part 64 - Implement sorting
In this video, we will discuss deleting multiple rows in an asp.net mvc application.
We will be using table tblEmployee for this demo. Please refer to Part 62, if you need SQL script to create and populate this table.
We want to provide a checkbox next to every row, to enable users to select multiple rows for deletion as shown below.
Step 1: Create an empty asp.net mvc 4 application.
Step 2: Generate ADO.NET entity data model from database using table tblEmployee. Change the entity name from tblEmployee to Employee. Save changes and build the application.
Step 3: Add HomeController with the following settings.
a) Controller name = HomeController
b) Template = Empty MVC controller
Setp 4: Add "Shared" folder under "Views", if it is not already present. Add "EditorTemplates" folder, under "Shared" folder. Right click on "EditorTemplates" folder and "Employee.cshtml" view with the following settings
View name = Employee
View engine = Razor
Create a strongly-typed view = checked
Model class = Employee (MVCDemo.Models)
Scaffold Template = Empty
and finally click "Add"
Step 5: Copy and paste the following code in Employee.cshtml view
@model MVCDemo.Models.Employee
<tr>
<td>
<input type="checkbox" name="employeeIdsToDelete" id="employeeIdsToDelete" value="@Model.ID" />
</td>
<td>
@Model.Name
</td>
<td>
@Model.Gender
</td>
<td>
@Model.Email
</td>
</tr>
Step 6: Copy and paste the following code in HomeController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MVCDemo.Models;
namespace MVCTest.Controllers
{
public class HomeController : Controller
{
private SampleDBContext db = new SampleDBContext();
public ActionResult Index()
{
return View(db.Employees.ToList());
}
[HttpPost]
public ActionResult Delete(IEnumerable<int> employeeIdsToDelete)
{
db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)).ToList().ForEach(db.Employees.DeleteObject);
db.SaveChanges();
return RedirectToAction("Index");
}
}
}
Setp 7: Right click on the Index() action and add "Index" view with the following settings.
View name = Index
View engine = Razor
Create a strongly-typed view = checked
Model class = Employee (MVCDemo.Models)
Scaffold Template = Empty
and finally click "Add"
Setp 8: Copy and paste the following code in Index.cshtml view
@model IEnumerable<MVCDemo.Models.Employee>
<div style="font-family:Arial">
<h2>Employee List</h2>
@using (Html.BeginForm("Delete", "Home", FormMethod.Post))
{
<table border="1">
<thead>
<tr>
<th>
Select
</th>
<th>
Name
</th>
<th>
Gender
</th>
<th>
Email
</th>
</tr>
</thead>
<tbody>
@Html.EditorForModel()
</tbody>
</table>
<input type="submit" value="Delete selected employees" />
}
</div>
In our next video, we will discuss providing a "SELECT ALL" checkbox to select and de-select all rows.
Part 62 - Implementing search functionality
Part 63 - Implement paging
Part 64 - Implement sorting
In this video, we will discuss deleting multiple rows in an asp.net mvc application.
We will be using table tblEmployee for this demo. Please refer to Part 62, if you need SQL script to create and populate this table.
We want to provide a checkbox next to every row, to enable users to select multiple rows for deletion as shown below.

Step 1: Create an empty asp.net mvc 4 application.
Step 2: Generate ADO.NET entity data model from database using table tblEmployee. Change the entity name from tblEmployee to Employee. Save changes and build the application.
Step 3: Add HomeController with the following settings.
a) Controller name = HomeController
b) Template = Empty MVC controller
Setp 4: Add "Shared" folder under "Views", if it is not already present. Add "EditorTemplates" folder, under "Shared" folder. Right click on "EditorTemplates" folder and "Employee.cshtml" view with the following settings
View name = Employee
View engine = Razor
Create a strongly-typed view = checked
Model class = Employee (MVCDemo.Models)
Scaffold Template = Empty
and finally click "Add"
Step 5: Copy and paste the following code in Employee.cshtml view
@model MVCDemo.Models.Employee
<tr>
<td>
<input type="checkbox" name="employeeIdsToDelete" id="employeeIdsToDelete" value="@Model.ID" />
</td>
<td>
@Model.Name
</td>
<td>
@Model.Gender
</td>
<td>
@Model.Email
</td>
</tr>
Step 6: Copy and paste the following code in HomeController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MVCDemo.Models;
namespace MVCTest.Controllers
{
public class HomeController : Controller
{
private SampleDBContext db = new SampleDBContext();
public ActionResult Index()
{
return View(db.Employees.ToList());
}
[HttpPost]
public ActionResult Delete(IEnumerable<int> employeeIdsToDelete)
{
db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)).ToList().ForEach(db.Employees.DeleteObject);
db.SaveChanges();
return RedirectToAction("Index");
}
}
}
Setp 7: Right click on the Index() action and add "Index" view with the following settings.
View name = Index
View engine = Razor
Create a strongly-typed view = checked
Model class = Employee (MVCDemo.Models)
Scaffold Template = Empty
and finally click "Add"
Setp 8: Copy and paste the following code in Index.cshtml view
@model IEnumerable<MVCDemo.Models.Employee>
<div style="font-family:Arial">
<h2>Employee List</h2>
@using (Html.BeginForm("Delete", "Home", FormMethod.Post))
{
<table border="1">
<thead>
<tr>
<th>
Select
</th>
<th>
Name
</th>
<th>
Gender
</th>
<th>
</th>
</tr>
</thead>
<tbody>
@Html.EditorForModel()
</tbody>
</table>
<input type="submit" value="Delete selected employees" />
}
</div>
In our next video, we will discuss providing a "SELECT ALL" checkbox to select and de-select all rows.
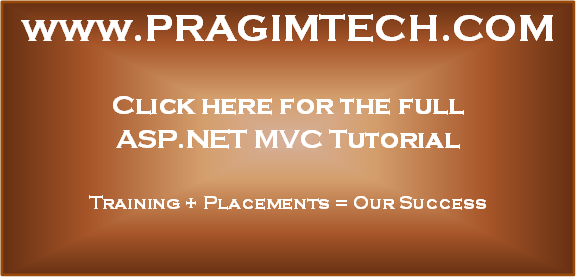
Thanks for this example, I have a similar situation and is very useful to know how to pass id's back to the controller.
ReplyDeleteBut I like to know if we can pass more data back to the controller not just id's to be deleted.
lets say the Employee table has a integer field called "AnnualLeaveDays" and we display that next to email. next to the "Delete Selected Employees" button if there is a textbox to enter integer, when user checks some rows to be deleted and then fills in a value how can we pass that to the controller?,
Please let me know if you have any ideas..
Thank you
Hi Sir,
ReplyDeleteIn the piece of code
db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)).ToList().ForEach(db.Employees.DeleteObject);
DeleteObject is not showing
hi alok you can see this website to enable db.Employee.DeleteObject
Deletehttp://msdn.microsoft.com/en-US/data/jj556581
Delete the .tt files in entity data model which you have added.
HI Alok,,
ReplyDeleteIt's bacouse you are using EF5 or above
U can use Remove insted of DeleteObject
Eg:.
List lst = db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)).ToList();
foreach (Employee item in lst)
{
db.Employees.Remove(item);
}
db.SaveChanges();
Employee emp = new Employee();
Deleteforeach (int id in employeeIdsToDelete)
{
emp = db.Employees.Find(id);
db.Employees.Remove(emp);
db.SaveChanges();
}
[HttpPost]
Delete[ActionName("MultiDelete")]
public ActionResult MultiDelete_Post(IEnumerable associateID)
{
(from associate in db.Associates
where associateID.Contains(associate.ID.ToString())
select associate).ToList().ForEach(x => db.Associates.Remove(x));
db.SaveChanges();
return View(db.Associates.ToList());
}
Thank you very much on these videos, for me it's very helpful!
ReplyDeleteI have one question, if someone can answer me: how to make check boxes to be saved when we move to another page,, e.g. I check one employee on page1, then I go to page2 and click another employee. How can it be achieved to remember seleted employee from page1?
i have executed all your mvc programs..but in this program i am getting error at DeleteObject..i have imported all packages...please help me solve this error as follows:
ReplyDeleteError 1 'System.Data.Entity.DbSet' does not contain a definition for 'DeleteObject' and no extension method 'DeleteObject' accepting a first argument of type 'System.Data.Entity.DbSet' could be found (are you missing a using directive or an assembly reference?) C:\Users\Amruta\Desktop\blazon\mvc programs\MvcMultipleDelete\MvcMultipleDelete\Controllers\HomeController.cs 23 107 MvcMultipleDelete
[HttpPost]
ReplyDeletepublic ActionResult Delete(IEnumerable employeeIdsToDelete)
{
db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)).ToList().ForEach(db.Employees.DeleteObject);
db.SaveChanges();
return RedirectToAction("Index");
}
in this code the parameter employeeIdsToDelete is collection for all ids then how it is recognizing checked ids
Severity Code Description Project File Line Suppression State
ReplyDeleteError CS1061 'DbSet' does not contain a definition for 'DeleteObject' and no accessible extension method 'DeleteObject' accepting a first argument of type 'DbSet' could be found (are you missing a using directive or an assembly reference?) MvcMultipleRecoredDelete E:\MvcPracticalVenkat\MvcMultipleRecoredDelete\MvcMultipleRecoredDelete\Controllers\HomeController.cs 23 Active
MVC5 Its Working These Code
ReplyDelete[HttpPost]
public ActionResult Delete(IEnumerable employeeIdsToDelete)
{
db.Employees.RemoveRange(db.Employees.Where(x => employeeIdsToDelete.Contains(x.ID)));
db.SaveChanges();
return RedirectToAction("Index");
}